Nprogress Loading
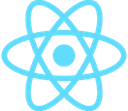
React Component for Nprogress Loading.
1
Install
nprogress
packageTerminal
npm i nprogress
# or
pnpm i nprogress
# or
yarn add nprogress
2
Create a
Progress
Componentcomponents/Progress.tsx
import NProgress from 'nprogress';
import "nprogress/nprogress.css";
import { useRouter } from 'next/router';
import { useEffect } from 'react';
// nprogrss config
NProgress.configure({
easing: "ease",
speed: 800,
showSpinner: false,
});
export default function Progress() {
const router = useRouter();
useEffect(() => {
const start = () => {
NProgress.start();
};
const end = () => {
NProgress.done();
};
router.events.on("routeChangeStart", start);
router.events.on("routeChangeComplete", end);
router.events.on("routeChangeError", end);
return () => {
router.events.off("routeChangeStart", start);
router.events.off("routeChangeComplete", end);
router.events.off("routeChangeError", end);
};
}, [router.events]);
return <></>;
}
3
Adding Some Custom Styling
styles.css
/* Nprogress bar Custom Styling (force) : STARTS */
#nprogress {
pointer-events: none;
}
#nprogress .bar {
background-color: rgba(0, 89, 255, 0.7) !important;
height: 3px !important;
}
.dark #nprogress .bar {
background: #fff !important;
}
#nprogress .peg {
box-shadow: none !important;
}
/* Nprogress bar Custom Styling (force) : ENDS */
4
Using the Component
I am using nprogress
in Next.js project. You can use directly in React as well.
pages/_app.tsx
import type { AppProps } from 'next/app';
import NProgress from '../components/nprogress';
export default function App({ Component, pageProps }: AppProps) {
return (
<>
<Component {...pageProps} />
<NProgress />
</>
);
}