Using Supabase Webhooks
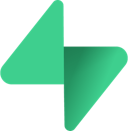
It shows how to use Supabase webhooks to revalidate pages.
Create Supabase Project
You need the following things
SUPABASE_URL
: Database URL.SUPABASE_KEY
: It is safe to be used in a browser context.
Steps
-
To get these go to Supabase and log in with your account.
-
Click on New Project and fill all the fields.
-
Click on Create New Project.
-
Go to the Settings page in the Dashboard.
-
Click API in the sidebar.
-
Find your API URL and anon key on this page.
-
Now you can Create table and start using it.
Webhooks
As I am using Supabase, It has a feature called webhooks which allow you to send real-time data from your database to another system whenever a table event occurs. So I am using it to revalidate my projects
and certificates
page. For that I am providing a custom secret value to verify that request is coming from authenticated source. Let's create webhook:
-
Go to webhooks page.
-
Click on Create a new hook
-
Enter the name of the function hook (example:
update_projects
) -
Choose your table from the dropdown list
-
Select events which will trigger this function hook
-
Now Choose POST method and enter the revalidate URL (request will be sent to this URL)
-
Then add two HTTP Params
secret
andrevalidateUrl
-
Now add this secret to your
env.local
and it will update the page when you made some changes to your supabase database. -
pages/api/revalidate.ts
is usingrevalidateUrl
to update the page with new data.
Creating pages/api/revalidate.ts
pages/api/revalidate.ts
import type { NextApiRequest, NextApiResponse } from "next";
interface ExtendedNextApiRequest extends NextApiRequest {
query: {
revalidateUrl: string;
secret: string;
};
}
export default async function handler(
req: ExtendedNextApiRequest,
res: NextApiResponse
) {
// Check for secret to confirm this is a valid request
if (req.query.secret !== process.env.REVALIDATE_SECRET) {
return res.status(401).json({
message:
"Invalid token alert! It looks like you're trying to sneak in without proper authorization. Please present a valid token or face rejection",
});
}
try {
// Regenerate the projects page
await res.revalidate(req.query.revalidateUrl);
return res.json({ revalidated: true });
} catch (err) {
// If there was an error, Next.js will continue
// to show the last successfully generated page
return res.status(500).send("Error revalidating");
}
}
Now, whenever you update your table the page will revalidate automatically.